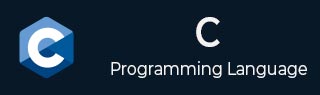
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Bit Fields in C
When we declare a struct or a union type, the size of the struct/union type variable depends on the individual size of its elements. Instead of the default memory size, you can set the size the bits to restrict the size. The specified size is called bit fields.
This is the syntax you would use to declare a bit field −
struct { data_type elem : width; };
Let's suppose your C program contains a number of TRUE/FALSE variables grouped in a structure called status, as follows −
struct { unsigned int widthValidated; unsigned int heightValidated; } status;
This structure requires 8 bytes of memory space but in actual, we are going to store either "0" or "1" in each of the variables. The C programming language offers a better way to utilize the memory space in such situations.
If you are using such variables inside a structure, then you can define the width of the variables which tells the C compiler that you are going to use only those many number of bytes. For example, the above structure can be re-written as follows −
struct { unsigned int widthValidated : 1; unsigned int heightValidated : 1; } status;
The above structure requires 4 bytes of memory space for the status variable, but only 2 bits will be used to store the values.
Example
If you will use up to 32 variables, each one with a width of 1 bit, then also the status structure will use 4 bytes. However, as soon as you have 33 variables, it will allocate the next slot of the memory and it will start using 8 bytes.
Let us check the following example to understand the concept −
#include <stdio.h> /* define simple structure */ struct { unsigned int widthValidated; unsigned int heightValidated; } status1; /* define a structure with bit fields */ struct { unsigned int widthValidated : 1; unsigned int heightValidated : 1; } status2; int main() { printf("Memory size occupied by status1: %d\n", sizeof(status1)); printf("Memory size occupied by status2: %d\n", sizeof(status2)); return 0; }
Output
When the above code is compiled and executed, it produces the following Output −
Memory size occupied by status1: 8 Memory size occupied by status2: 4
Bit Field Declaration
The declaration of a bit-field has the following form inside a structure −
struct { type [member_name] : width ; };
The following table describes the variable elements of a bit field −
Element | Description |
---|---|
type | An integer type that determines how a bit-field's value is interpreted. The type may be int, signed int, or unsigned int. |
member_name | The name of the bit-field. |
width | Number of bits in the bit-field. "width" must be less than or equal to bit width of specified type. |
The variables defined with a predefined width are called bit fields. A bit field can hold more than a single bit; for example, if you need a variable to store a value from 0 to 7, then you can define a bit field with a width of 3 bits, as follows −
struct { unsigned int age : 3; } Age;
The above structure definition instructs the C compiler that the variable "Age" is going to use only 3 bits to store the value. If you try to use more than 3 bits, then it will not allow you to do so.
Example
Let us try the following example −
#include <stdio.h> struct { unsigned int age : 3; } Age; int main() { Age.age = 4; printf("Sizeof(Age): %d\n", sizeof(Age)); printf("Age.age: %d\n", Age.age); Age.age = 7; printf("Age.age : %d\n", Age.age); Age.age = 8; printf("Age.age : %d\n", Age.age); return 0; }
Output
When the above code is compiled, it will compile with a warning −
warning: unsigned conversion from 'int' to 'unsigned char:3' changes value from '8' to '0' [-Woverflow]|
And, when executed, it will produce the following output −
Sizeof(Age): 4 Age.age: 4 Age.age: 7 Age.age: 0
You can use Bit Fields in situations where the available storage is limited. Bit Fields also prove efficient when devices transmit status or information encoded into multiple bits. Whenever certain encryption programs need to access the bits within a byte, bit fields are used to define the data structure.
To Continue Learning Please Login